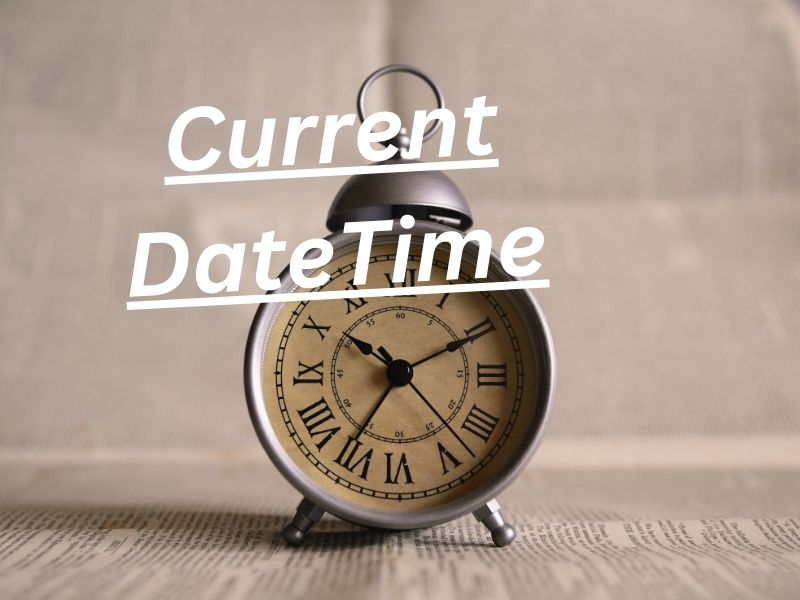
Through the use of the datetime module in Python, it is possible to obtain the current time. To be more specific, you may retrieve a datetime object that represents the current date and time by utilizing the datetime.now() function. One example is as follows:
from datetime import datetime
# Get the current date and time
current_time = datetime.now()
print("Current Time:", current_time)
For situations in which you require only the current time and not the date information, you can make use of the time class that is contained within the datetime module:
from datetime import datetime
# Get the current time
current_time = datetime.now().time()
print("Current Time:", current_time)
Specifically, the current_time will be represented by the time object known as current_time in this scenario.
If you wish to format the time as a string in a certain manner, you can use the strftime method to format the datetime or time object. This method is included in the Time class.
from datetime import datetime
# Get the current time
current_time = datetime.now().time()
# Format the time as a string
formatted_time = current_time.strftime("%H:%M:%S")
print("Formatted Time:", formatted_time)
In the example above, "%H:%M:%S"
is the format string, where %H
represents the hour (00-23), %M
represents the minute (00-59), and %S
represents the second (00-59). Adjust the format string as needed to match your requirements.
Different ways
In Python, there are several ways to get the current date and time. Here are some common methods using different modules:
1. Using datetime
module:
from datetime import datetime
# Get current date and time
current_datetime = datetime.now()
print("Current Date and Time:", current_datetime)
2. Using date
and time
classes from datetime
module:
from datetime import date, time, datetime
# Get current date
current_date = date.today()
print("Current Date:", current_date)
# Get current time
current_time = datetime.now().time()
print("Current Time:", current_time)
3. Using time
module:
import time
# Get current time as seconds since the epoch
current_epoch_time = time.time()
print("Current Epoch Time:", current_epoch_time)
# Get current time in a readable format
current_readable_time = time.ctime()
print("Current Readable Time:", current_readable_time)
4. Using calendar
module for date-related information:
import calendar
# Get current date information
current_date_info = calendar.timegm(time.gmtime())
print("Current Date Information:", current_date_info)
Pick the approach that caters to your requirements the most effectively. Due to the fact that it is both flexible and thorough in its functionality, the datetime module is frequently the one that is utilized the most. Both the time module and the calendar module are capable of providing additional information that is connected to dates. The time module is primarily focused on time-related operations.