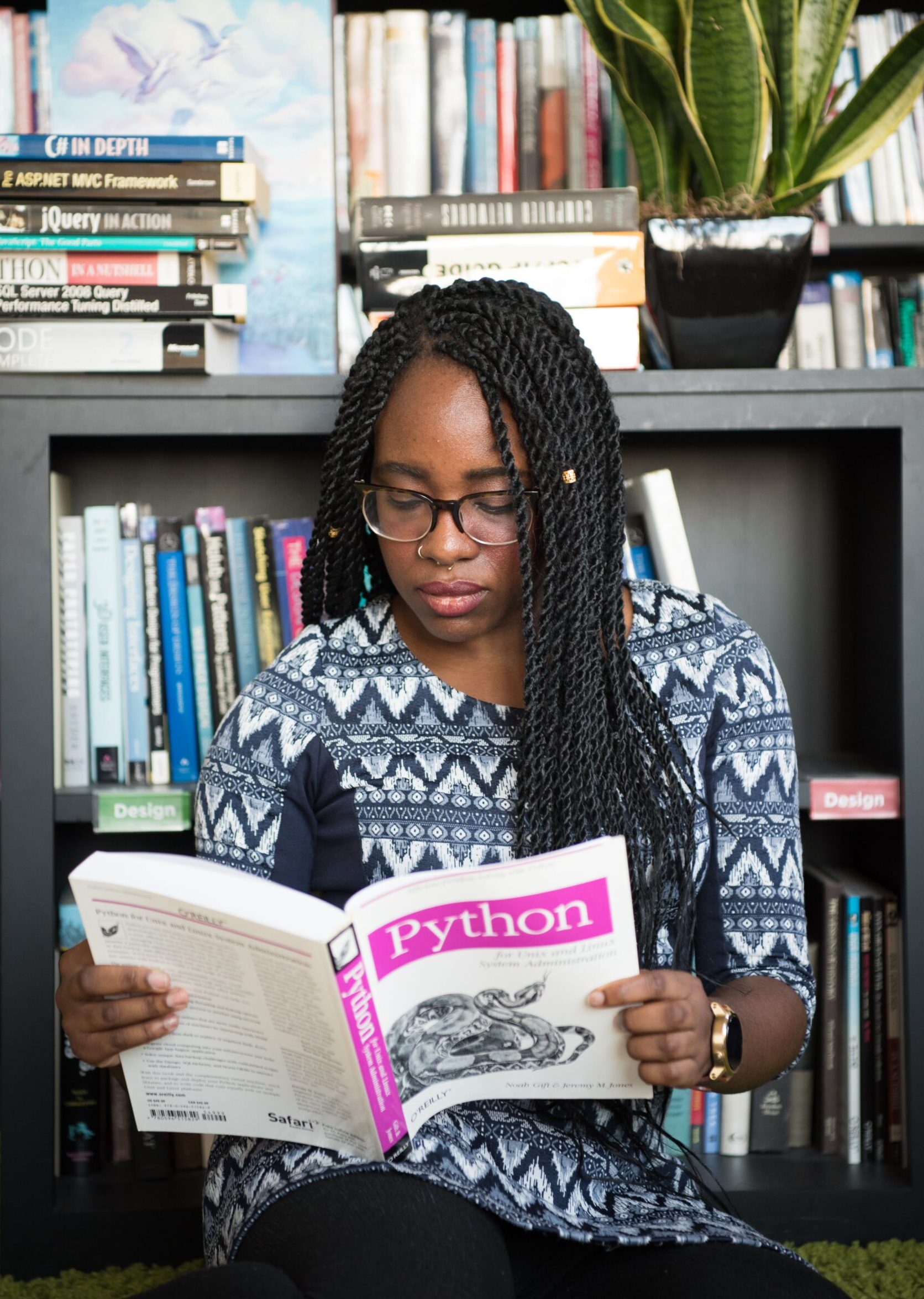
What does the “yield” keyword do in Python?
For the purpose of generating a series of values in Python, the yield keyword is used within the context of a generator function. When the yield statement is included in a function, the function is transformed into a generator. Therefore, invoking the function that generates the function results in the ...
Read moreHow do I get the current time?
Through the use of the datetime module in Python, it is possible to obtain the current time. To be more specific, you may retrieve a datetime object that represents the current date and time by utilizing the datetime.now() function. One example is as follows: For situations in which you require ...
Read moreTop 100 Python Interview Questions and Answers
Q1. What is the difference between list and tuples in Python? LIST TUPLES Lists are mutable i.e they can be edited. Tuples are immutable (tuples are lists which can’t be edited) Lists are slower than tuples. Tuples are faster than list. Syntax: list_1 = [10, ‘Chelsea’, 20] Syntax: tup_1 = ...
Read moreHow do I sort a dictionary by value?
In order to sort a dictionary based on its values in Python, the elements of the dictionary must first be converted into a list of tuples, with each tuple containing a key-value pair associated with it. After that, you may use the sorted function on this list, and you can ...
Read moreWhat does if __name__ == “__main__”: do?
When a Python script is being executed as the main program or imported as a module into another script, the special variable ‘name‘ is used to identify the execution mode. It is usual practice to use the expression “if name == “main“:” to verify that the Python script is being ...
Read moreBeautiful Soup in Python
Beautiful Soup is a popular Python library used for web scraping purposes to pull the data out of HTML and XML files. It creates a parse tree that can be used to extract data in a hierarchical and more readable manner. Installation To start using Beautiful Soup, you need to ...
Read morePytest Framework in Python
Pytest is a popular testing framework in Python that allows for simple unit tests as well as complex functional testing. It follows a no-boilerplate philosophy, which makes your test code concise and easy to read. With pytest, you can ensure the code behaves as expected and make code changes confidently. ...
Read moreFlask in Python
Flask is a micro web framework written in Python. It is classified as a “micro” framework because it does not require particular tools or libraries, nor does it have a specific pattern for building applications, like Django’s Model-View-Controller (MVC) pattern. However, this does not mean Flask is limited; rather, it ...
Read moreHow to send WhatsApp messages using Python ?
WhatsApp does not provide an official public API for sending messages or managing chats for regular accounts. The official API provided by WhatsApp is the WhatsApp Business API, which is primarily aimed at medium and large businesses to manage communication with their customers. However, there are several third-party libraries and ...
Read moreTop Python Interview Questions
Here are some common Python interview questions that span a range of topics: Basic Questions General Python Knowledge Data Structures Object-Oriented Programming Web Development Testing Concurrency and Parallelism File Handling Error and Exception Handling Python Libraries and Frameworks Python 2 vs Python 3 PEP 8 and Code Style Advance Questions ...
Read more